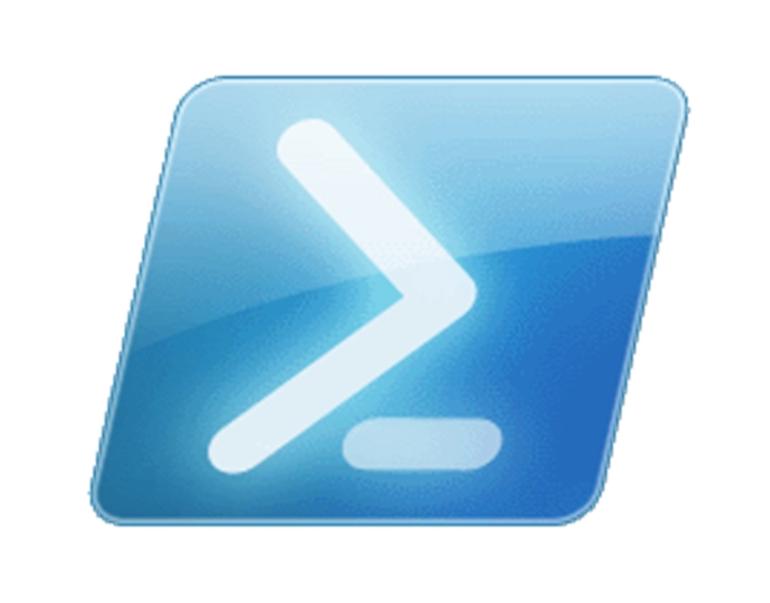
I recently had a request to create a replica of the company AD structure in a lab environment for testing with a new software product.
The task involves the exporting and importing of the OUs and groups used in the production Active directory to the lab.
Of course doing this manually would take hours in a large environment and be prone to human error. I had a few issues creating the right structure to export because the DistinguishedName contains the name of the group it needs to be removed from the list. I’ve managed to do this with regex.
To export the list of OUs I created the following. Essentially it builds a list of the OUs using the Get-ADOrganizationalUnit command. Next I use a foreach loop to isolate the values line by line and remove the first part of the DistinguishedName value that contains the OU name. Lastly, I use the New-Object command to create a hash table with the values I want and export this to a csv file.
import-module activedirectory
$OUlist = Get-ADOrganizationalUnit -Filter * | select name,DistinguishedName
foreach ($OU in $OUlist){
$name = $OU | Select-Object -ExpandProperty name
$DistinguishedName = $OU | Select-Object @{ Name = "ParentOU"; Expression = {[regex]::Match($_.distinguishedname,",(.*)").Groups[1].Value}}
$DistinguishedName = $DistinguishedName.ParentOU
New-Object -TypeName psobject -Property @{
name = $name
ParentOU = $DistinguishedName
} | Select-Object name,ParentOU | Export-csv -path C:\temp\ADOrganizationalUnitsexport.csv -NoTypeInformation -Append}
The CSV file can now be copied from the production environment to the lab environment. If you have a different domain name in your lab than your production, simply open the exported file in notepad and use the Replace function to resolve this.
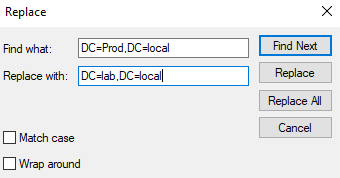
Once you’ve copied the export file to the lab use the following foreach loop to create all the OUs taking advantage of the New-ADOrganizationalUnit command.
import-module activedirectory
$OUlist = import-csv C:\temp\ADOrganizationalUnitsexport.csv
foreach ($OU in $OUlist)
{
Try {
Write-Host "Processing OU Object " $OU.name
New-ADOrganizationalUnit -Name $OU.name -Path $OU.ParentOU
}
Catch {
Write-Host "Already exists or error found..:" $_ -ForegroundColor Red
}
}
Great this worked! Now let’s try the same thing with AD groups using the Get-ADgroup command.
Import-Module ActiveDirectory
$grouplist = Get-ADgroup -Filter * -Properties groupscope,description,DistinguishedName| select samaccountname,groupscope,description,DistinguishedName | Sort-Object -Property Name
foreach ($group in $grouplist){
$name = $group | Select-Object -ExpandProperty samaccountname
$groupscope = $group | Select-Object -ExpandProperty groupscope
$description = $group | Select-Object -ExpandProperty description
$DistinguishedName = $group | Select-Object @{ Name = "ParentOU"; Expression = {[regex]::Match($_.distinguishedname,",(.*)").Groups[1].Value}}
$DistinguishedName = $DistinguishedName.ParentOU
New-Object -TypeName psobject -Property @{
samaccountname = $name
groupscope = $groupscope
description = $description
ParentOU = $DistinguishedName
} | Select-Object samaccountname,groupscope,description,ParentOU | Export-csv -path C:\temp\ADGroups.csv -NoTypeInformation -Append}
Copy the export the same as before to the lab. Now it’s time to create the groups in the lab. This is gone with a new-adgroup.
$groups=Import-csv C:\temp\ADGroups.csv
ForEach($group In $groups){
Try {
New-ADGroup -Name $group.samaccountname -GroupScope $group.Groupscope -Path $group.ParentOU -description $group.description
}
catch {
Write-Host "Group $($Group.samaccountname) was not created!" $Error[0]
}
}
That’s it. The OU and AD groups are now replicated in the lab. The export CSV can now be removed or stored somewhere else as a temple.